Every new release of C# is like Christmas to me. New features and syntactic sugar improvements feel like presents underneath a christmas tree. As soon as possible I jump on the opportunity to utilize those to make my code better (without sacrificing readability of course!).
Even though Sitecore supports .NET Framework up to the most recent 4.8 version, you will encounter errors when trying to use features introduced in C# 6 and higher in a view. To illustrate this, I created a simple rendering:
@{
var placeholder = RenderingContext.CurrentOrNull?.Rendering.Placeholder;
}
<div>Placeholder: @placeholder</div>
At line #2 I made use of the null-conditional operator (aka the Elvis operator) which was introduced in C# 6. I would be shocked if there's anybody not already familiar with it, but if you are, look up the documentation and make your life better.
After placing the component onto a page I got this:
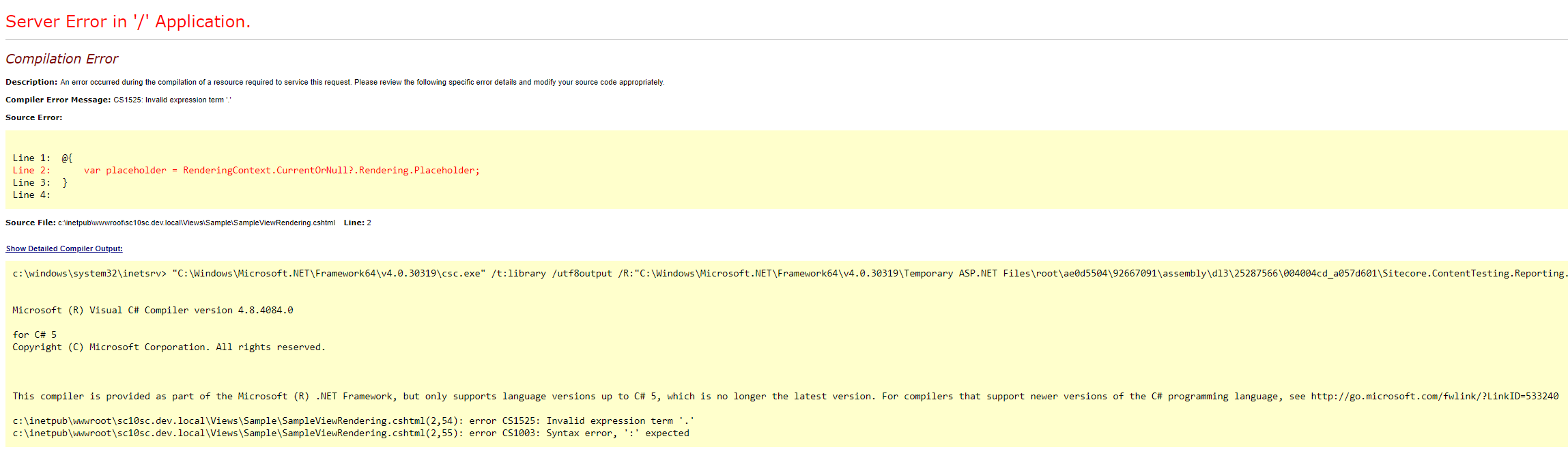
The problem is that Razor views are compiled at runtime, and runtime compilation is handled by CodeDOM providers. The one utilised by MVC 5 supports only C# 5.0.
But fear not, this can be fixed. Although, you will not be able to go higher than C# 7.3 with .NET Framework, that's still a big improvement over C# 5. You will be able to use features such as the aforementioned null-coalescing operator, but also string interpolation, pattern matching, tuples and many more.
To add support for newer C# versions, the .NET Compiler Platform (aka Roslyn) has to be added to your solution. The first step is to simply install the Microsoft.CodeDom.Providers.DotNetCompilerPlatform
package to your project using NuGet. You might notice that the following entries were added to the Web.config
of the project:
<system.codedom>
<compilers>
<compiler extension=".cs" language="c#;cs;csharp" warningLevel="4" compilerOptions="/langversion:7.3 /nowarn:1659;1699;1701;612;618" type="Microsoft.CodeDom.Providers.DotNetCompilerPlatform.CSharpCodeProvider, Microsoft.CodeDom.Providers.DotNetCompilerPlatform, Version=3.6.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" />
<compiler extension=".vb" language="vb;vbs;visualbasic;vbscript" warningLevel="4" compilerOptions="/langversion:default /nowarn:41008,40000,40008 /define:_MYTYPE=\"Web\" /optionInfer+" type="Microsoft.CodeDom.Providers.DotNetCompilerPlatform.VBCodeProvider, Microsoft.CodeDom.Providers.DotNetCompilerPlatform, Version=3.6.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" />
</compilers>
</system.codedom>
Now, Sitecore comes with its own Web.config
and the above fragment needs to be added to it. The best practice is to use config transformations on the original file. Simply add xdt:Transform="Insert"
to the <system.codedom>
element and put it in the transform file:
<system.codedom xdt:Transform="Insert">
<compilers>
<compiler extension=".cs" language="c#;cs;csharp" warningLevel="4" compilerOptions="/langversion:7.3 /nowarn:1659;1699;1701;612;618" type="Microsoft.CodeDom.Providers.DotNetCompilerPlatform.CSharpCodeProvider, Microsoft.CodeDom.Providers.DotNetCompilerPlatform, Version=3.6.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" />
<compiler extension=".vb" language="vb;vbs;visualbasic;vbscript" warningLevel="4" compilerOptions="/langversion:default /nowarn:41008,40000,40008 /define:_MYTYPE=\"Web\" /optionInfer+" type="Microsoft.CodeDom.Providers.DotNetCompilerPlatform.VBCodeProvider, Microsoft.CodeDom.Providers.DotNetCompilerPlatform, Version=3.6.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" />
</compilers>
</system.codedom>
And that's all! After deploying the Roslyn compiler and the updated Web.config
file, the rendering works as expected:

One more thing - using the newest version of the Roslyn compiler might also give a huge performance boost to the startup of your Sitecore solution! That's what I call a win-win scenario!
Cover photo by Daniel Mayo on Unsplash